Environment | |
---|---|
UnrealEngine | branch: 5.0 |
Visual Studio 2022 | version: 17.0.4 |
Windows 11 Pro | build: 22000.493 |
Overview
Sometimes, you might need to rename your project in some reasons.
- Just you may be bored with that name.
- To unify the name same with your team name.
- Due to change on design of game…etc.
Unfortunately, in those situations, UnrealEngine does not provide any feature to rename your project.
So, in this post, we gonna find out how to rename your project manually.
Prerequisites
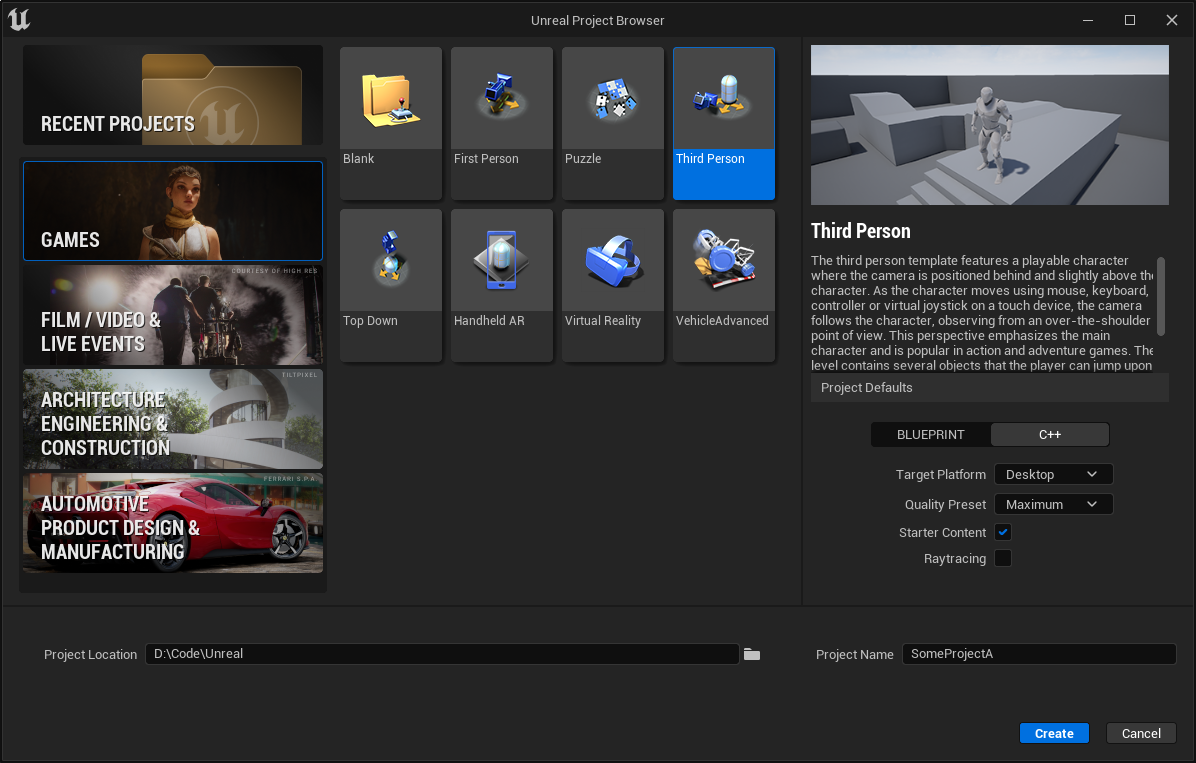
Suppose we have a project created from template Third Person
with options above.
1 | [ProjectRoot]/Source/SomeProjectA/SomeProjectACharacter.h |
After the project created, make a simple function GetSomeString()
in the character class, which returns some string. We will try to migrate the function for example later in this post. Now, build editor with the combo Development Editor + Win64
and run it.
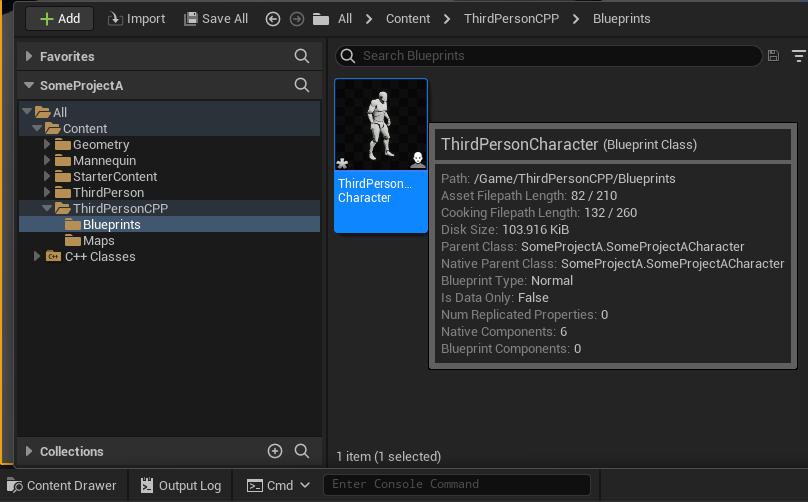
You can find a character blueprint created from template in the Content/ThirdPersonCPP/Blueprints/ThirdPersonCharacter
. Furthermore, the blueprint has a parent class as the cpp class SomeProjectACharacter
. It means that the blueprint can use the function we have just made.
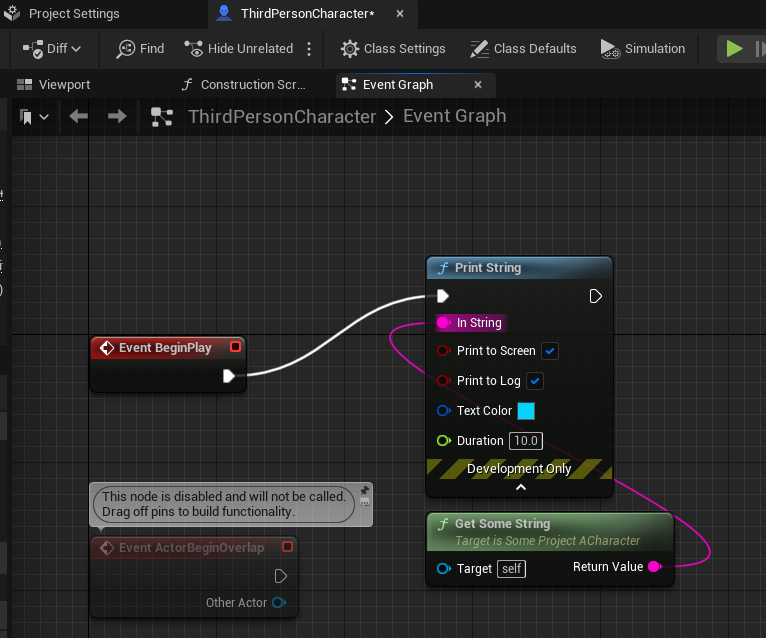
I think it would be proper to print that string when character spawned. Make some blueprint nodes for printing that string. Now, compile and save it.
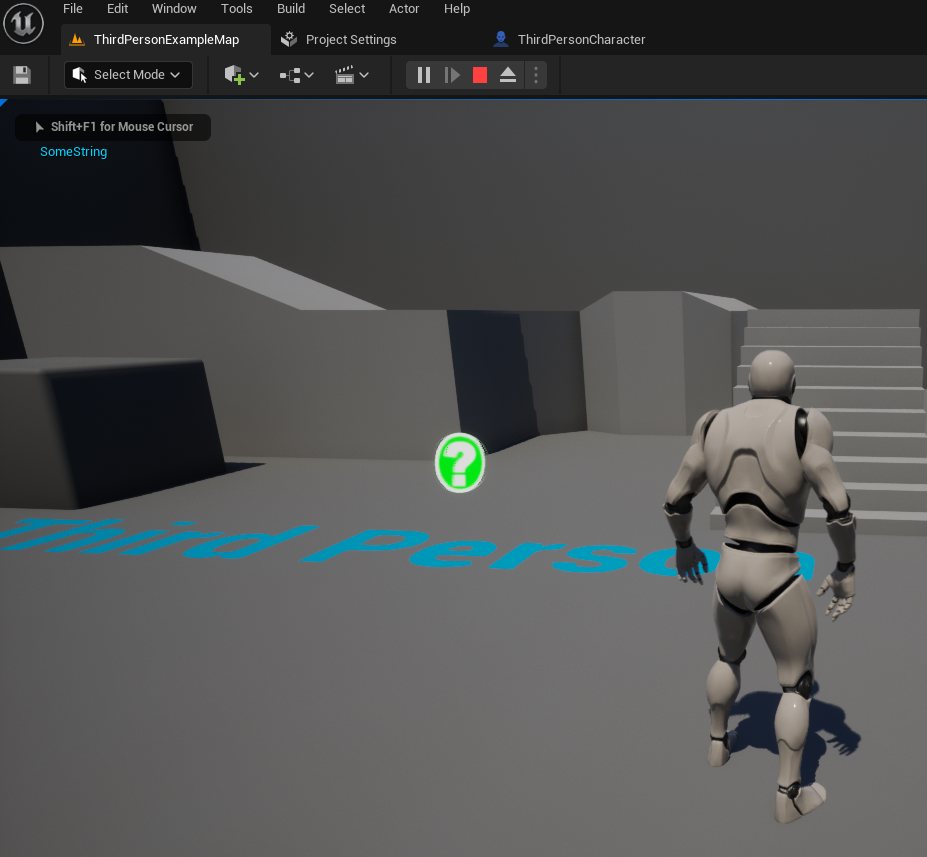
Check it works out. You should be able to see that string SomeString
through the screen. Great.
1 | .../SomeProjectA> git log |
I setup the project directory as git repository to clarify what is changed. You do not have to follow this, it is optional. But, you should prepare a gitignore fits in UnrealEngine if you want to follow this. (For example, https://github.com/github/gitignore/blob/main/UnrealEngine.gitignore)
We are all prepared, and let us change the name of project from SomeProjectA
into OtherProjectB
.
Step #1; Clean-up
First of all, we should remove some files. Some files and folders are generated by other files, so we do not have to care about that files would be generated later. Thus, we would better remove those files or folders listed below:
.vs/
Binaries/
DerivedDataCache/
Intermediate/
Saved/
[ProjectName].sln
You can check if the files or folders are generated. Remove them and generate VisualStudio project files. Then, files and folders related to VisualStudio would be generated. And you can build your project from VisualStudio project. After all, you will see the files and folders listed above are restored.
Plus, that is why gitignore for UnrealEngine contains those files or folders. We do not need them to be version-controlled.
Step #2; Change contents of files
Now it is time to rename the project. There are some files usually contain the name of project in its contents. Therefore, we should change that part of contents. In this goal, we will manipulate the files like…
- all of files in
Config/
.ini
files such asDefaultEngine.ini
- all of files in
Source/
[ProjectName].uproject
Maybe there some files contain the name of project in the folder Content/
. Such as an absolute path of media file, and a blueprint class inherites a cpp class whose name contains the name of project. However, basically the files in Content/
are binary type. So, manipulating its contents as text might not ensure a result we expect. In worst case, the manipulation could break some references between blueprints. That is why we handle only the files of text type in this step.
By the way, I recommend you to use notepad++ when manipulating multiple text files, and I will use that in this post. It is open source and provides powerful features. The tool supports Windows and you can install it for ease. You do not have to install it, but I will show an example based on the tool.
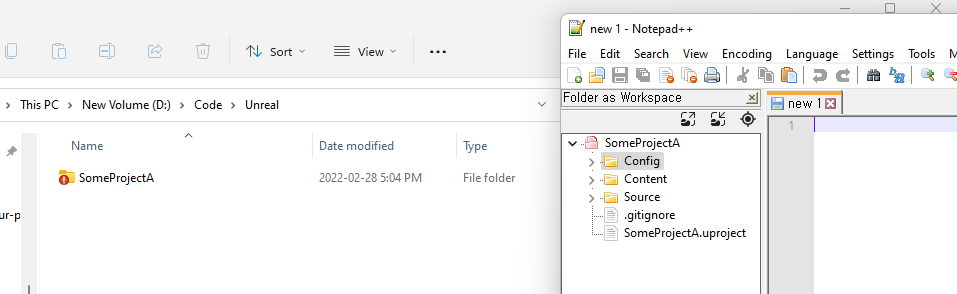
Open the notepad++ and drag your project folder from file explorer into notepad++. Now notepad++ would show the folder as list view at the left sidebar.
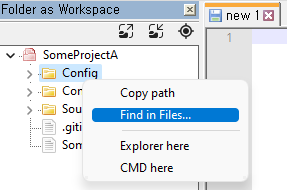
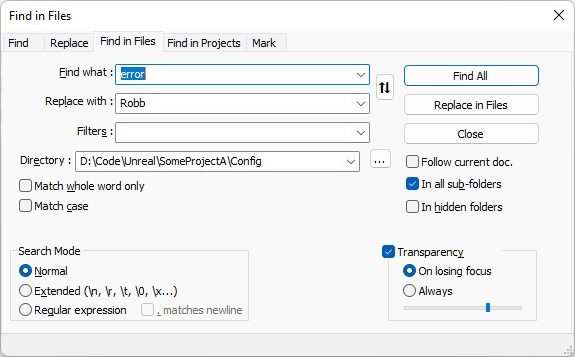
Right click on Config/
and select Find in Files...
. Then, a dialog for search would appear.
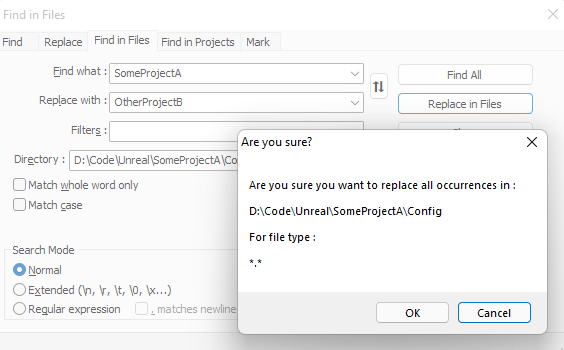
Click the tab Find in Files
and type SomeProjectA
and OtherProjectB
respectively at Find what
and Replace with
. After that, click Replace in Files
.
Repeat the steps on Source/
folder.
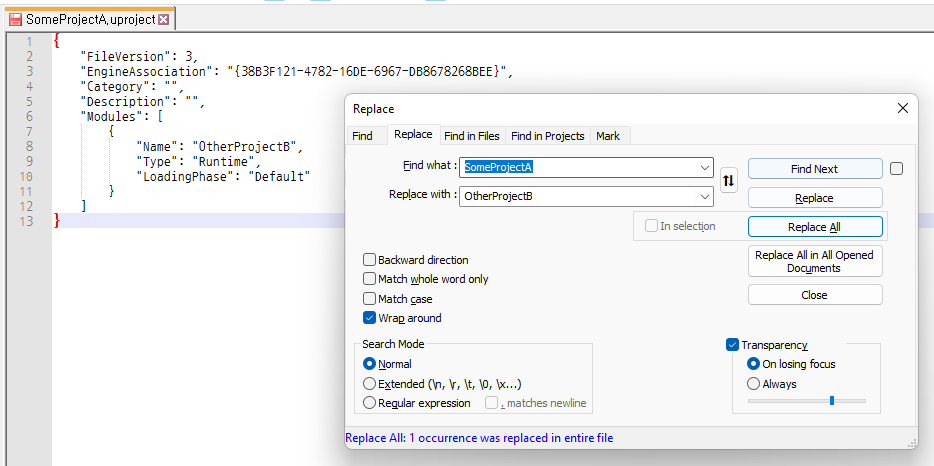
Open the .uproject
file and Replace in similar manner.
1 | .../SomeProjectA> git status |
We can see some files changed. Now the contents of file get ready.
Step #3; Change name of files
We have changed the contents of files. Next, let us change the name of files. This also works whole project without Content/
folder with the same reason I mentioned.
Open a powershell prompt and type the command like below:
1 | %ProjectRoot% > Get-ChildItem -Recurse -Path Config/* | Rename-Item -NewName { $_.Name.replace("SomeProjectA","OtherProjectB") } |
This will change the name of all files in Config/
folder.
1 | %ProjectRoot% > Get-ChildItem -Recurse -Path Source/* | Rename-Item -NewName { $_.Name.replace("SomeProjectA","OtherProjectB") } |
Apply this on Source/
folder, too. After that, change the name of .uproject
file and [ProjectRoot]
folder manually.
1 | .../OtherProjectB> git status |
Check the result with git status
again.
Step #4; Redirect blueprints
Generating VisualStudio project files okay. Building editor on VisualStudio project okay. But, there is one last task to do.
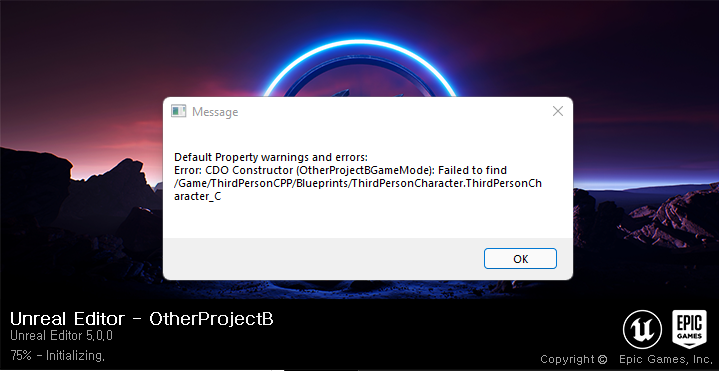
We skipped blueprint files in previous steps. But, some blueprint assets could try to use old cpp classes or codes. Therefore, you will see the dialog while opening the editor. The CDO has been broken.
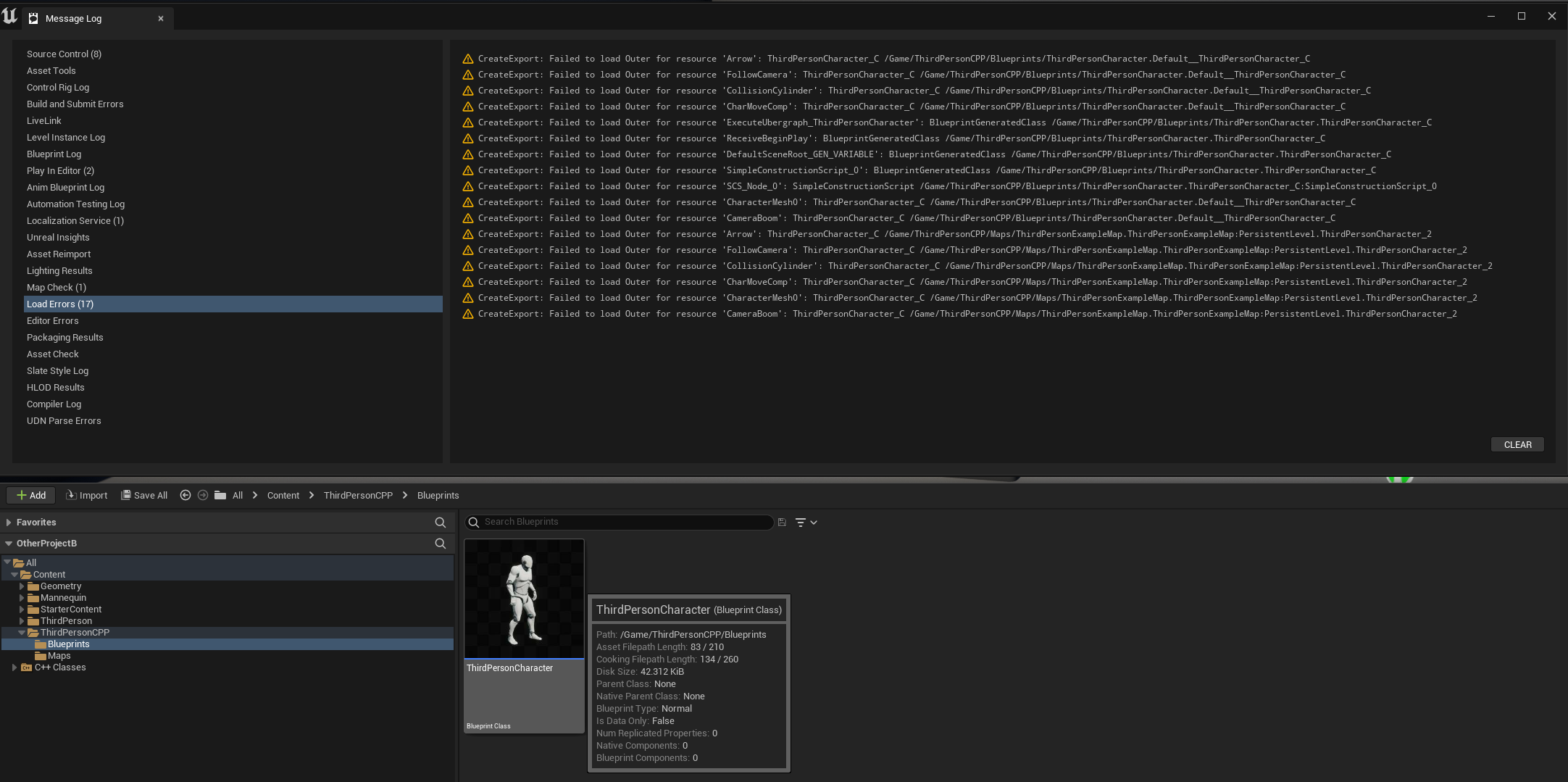
Some of blueprint classes lost their parent cpp class or get broken. Especially, the blueprint class ThirdPersonCharacter
was disconnected with its parent, old cpp class SomeProjectACharacter
. We need to fix it.
1 | // DefaultEngine.ini |
Fortunately, UnrealEngine provides redirecting blueprint classes. You can set the redirection settings in DefaultEngine.ini
. I have set the settings like above, and engine will redirect SomeProjectA
things into OtherProjectB
things. You should create more settings if you need. Because the example settings are from template and your project may have more classes whose CDO broken.
After setting up the redirection, remove Binaries/
+ DerivedDataCache/
+ Saved/
folders. And repeat build the editor.
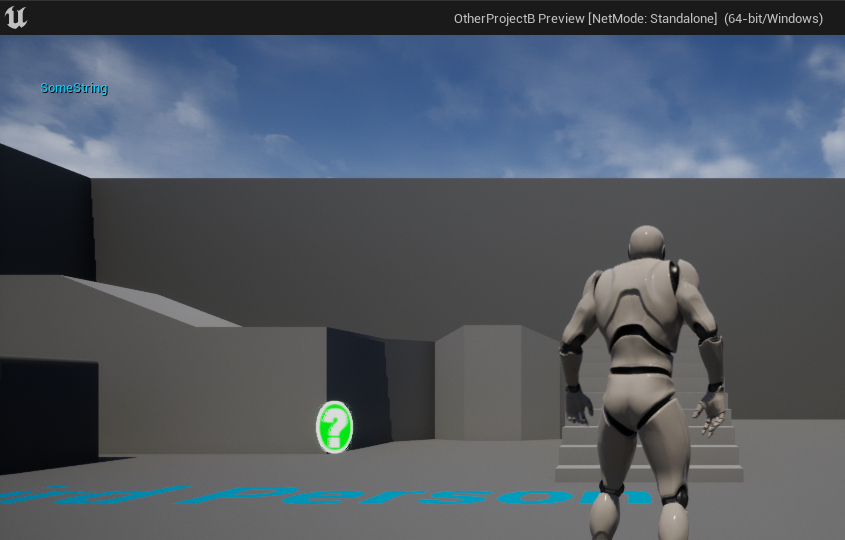
Finally we meet again ! The string SomeString
was the text we prepared. We have done renaming a project and restoring whole project.